A push notification is a message that pops up on a mobile device. App publishers can send them at any time; users don't have to be in the app or using their devices to receive them.
Firebase Cloud Messaging (FCM) is a cross-platform service that handles the sending, routing, and queueing of messages between server applications and mobile client apps. FCM is the successor to Google Cloud Messaging (GCM), and it is built on Google Play Services. https://docs.microsoft.com/en-us/xamarin/android/data-cloud/google-messaging/firebase-cloud-messaging
If you’ve got a mobile application, then you need to know what push notifications are. So let's get started.
Setting up Firebase Cloud Messaging
Before you can use FCM services in your app, you must create a new project via the Firebase Console. Use the following steps to create a Firebase Cloud Messaging project for your app:
Step: 1: Sign into the with your Google account (i.e., your Gmail address) and click Add Project:
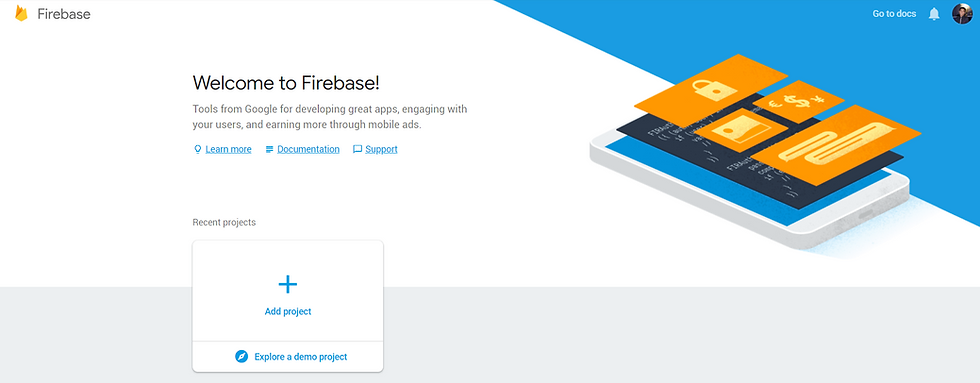
Step 2: In the Add a project dialog, enter the name of your project and click Create Project. In the following example, a new project called MyAwesomeFCM is created:
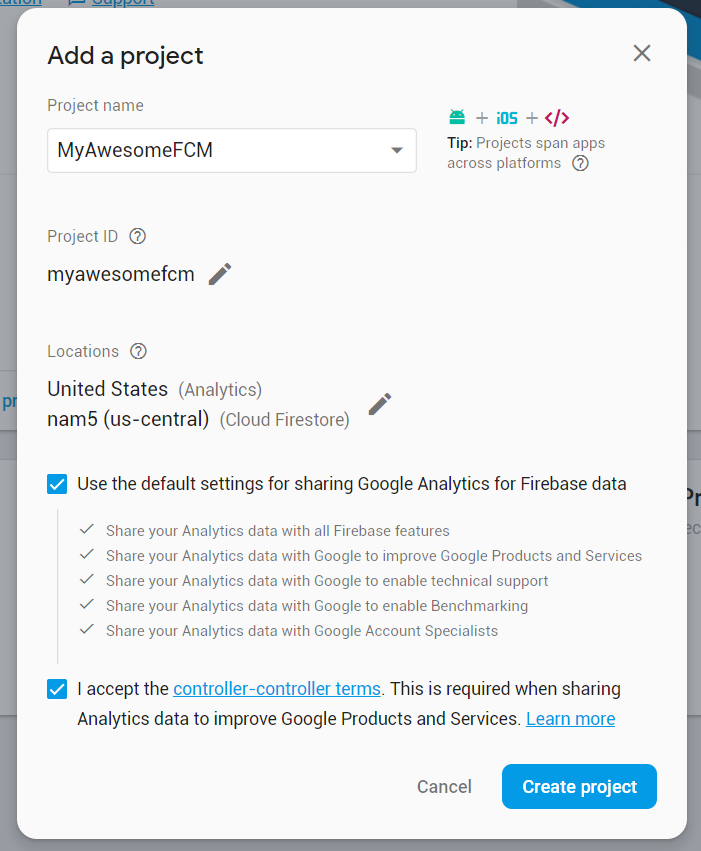
Step 3: In the Firebase Console Overview, click the Android icon:
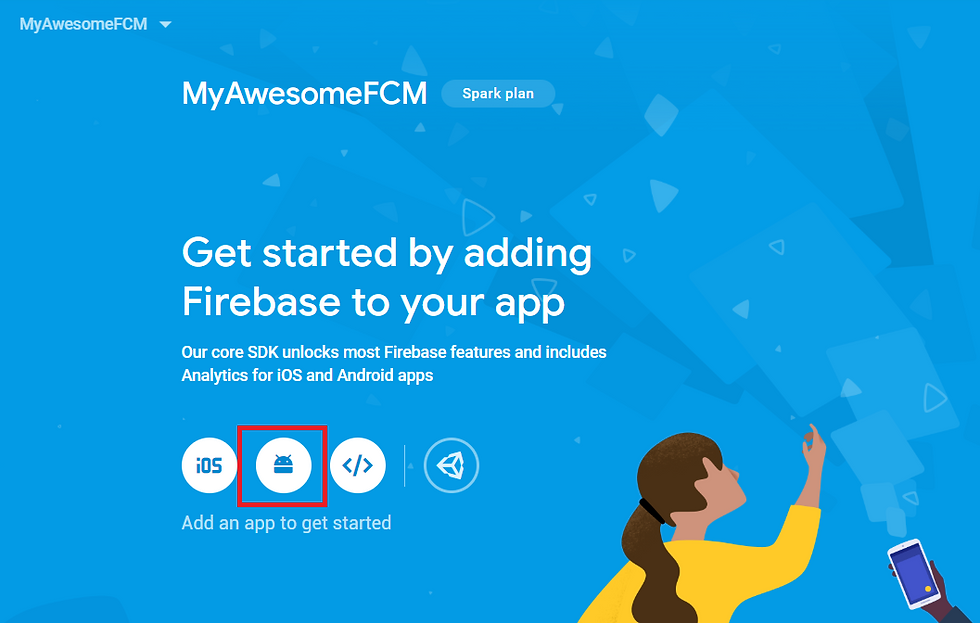
Step 4: In the next screen, enter the package name of your app. In this example, the package name is com.xamarin.myawesomefcm. This value must match the package name of your Android app. An app nickname can also be entered in the App nickname field. Then click Register app:
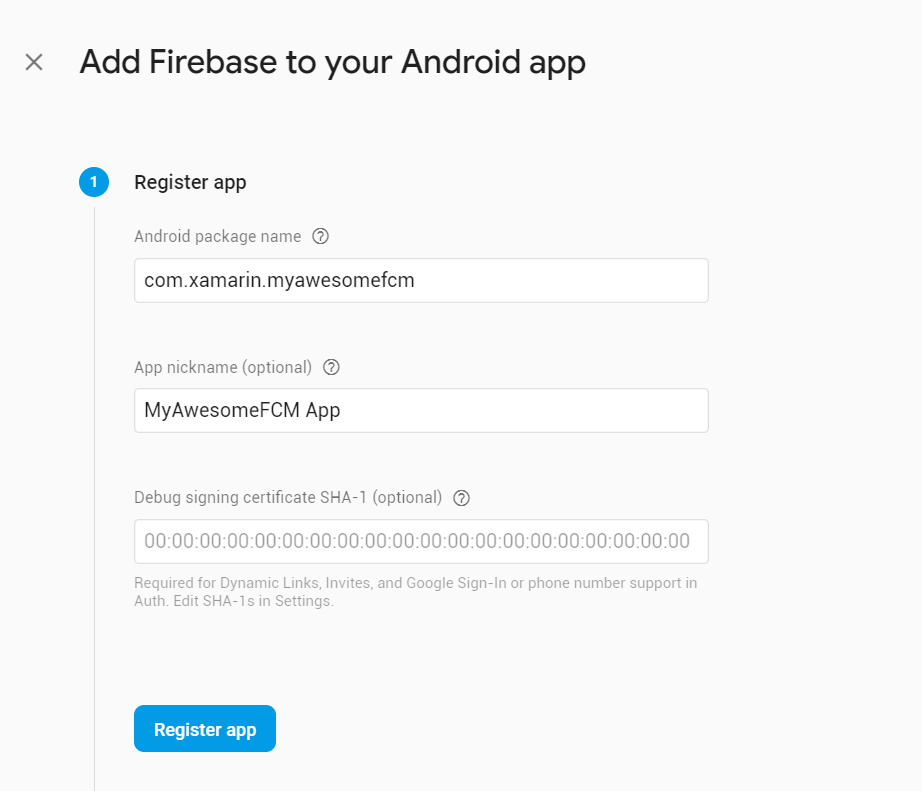
Step 5: Start an app project. To begin, create a new empty Mobile App (Xamarin.Forms) project called XFormsFCM. After the new app is created, the next step is to set the package name and install several NuGet packages that will be used for communication with FCM.
Set the Package name using the package name you specified in Firebase Cloud Messaging:

To receive messages from FCM, the Xamarin Firebase - Messaging NuGet package must be added to the Android project:
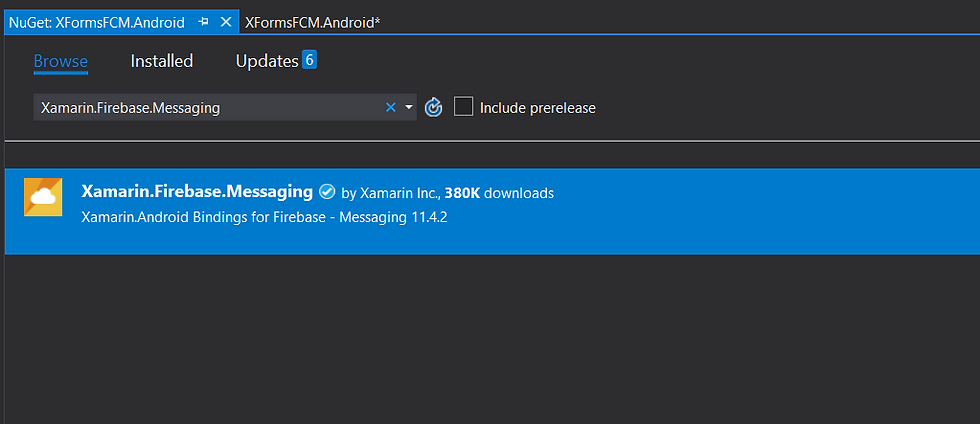
Step 6: Add a service that extends FirebaseInstanceIdService to handle the creation, rotation, and updating of Firebase registration tokens. The FirebaseInstanceIdService service is required for FCM to be able to send messages to the device. When the FirebaseInstanceIdService service is added to the client app, the app will automatically receive FCM messages and display them as notifications whenever the app is backgrounded.
Edit AndroidManifest.xml and insert the following <receiver> elements into the <application> section:
Step 7: Add a new file called MyFirebaseIIDService.cs and replace its template code with the following:
Step 8: Edit MainActivity.cs and insert FirebaseOptions just before the LoadApplication method:
You will get the App ID, API Key and the Sender ID in you Firebase Project Settings.
Step 9: Build and run the app. A message like the following should be displayed in the IDE output window:
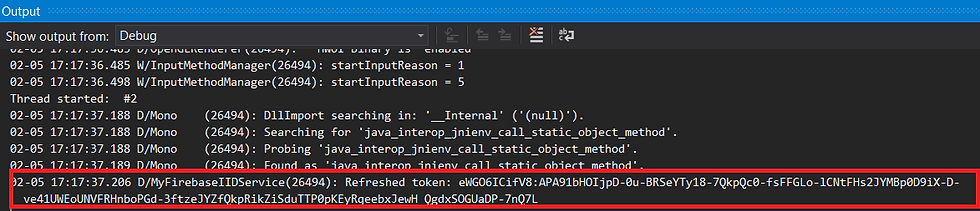
Step 10: Add a new file called MyFirebaseMessagingService.cs and replace its template code with the following:
Step 11: Add a local notification sender. Edit MyFirebaseMessagingService.cs and insert SendNotification method just after the OnMessageReceived method:
Edit OnMessageReceived method:
Send a message
Uninstall the app, rebuild it, run it again, and follow these steps to send message:
In the Firebase Console, click Cloud Messaging.
Click Send your first message.
On the Compose notification page, enter the notification text and click Test on device.
Copy the token string from the IDE output window and paste it into the FCM registration token field.
Ensure that the app is running in the foreground, then click Test.
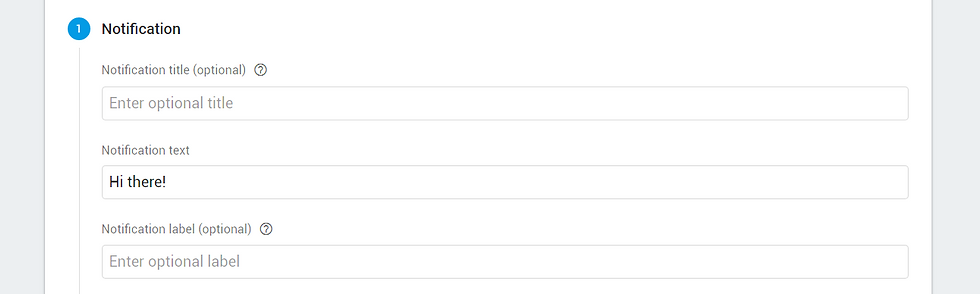
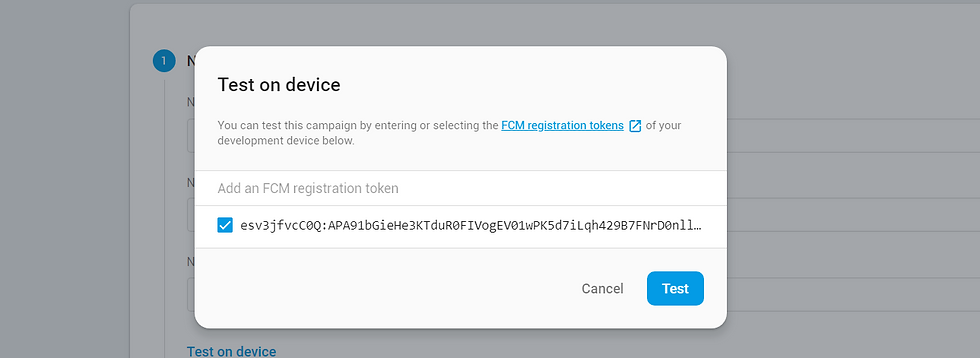
The message is packaged in a new notification – the notification icon appears in the notification tray while the app is running in the foreground.
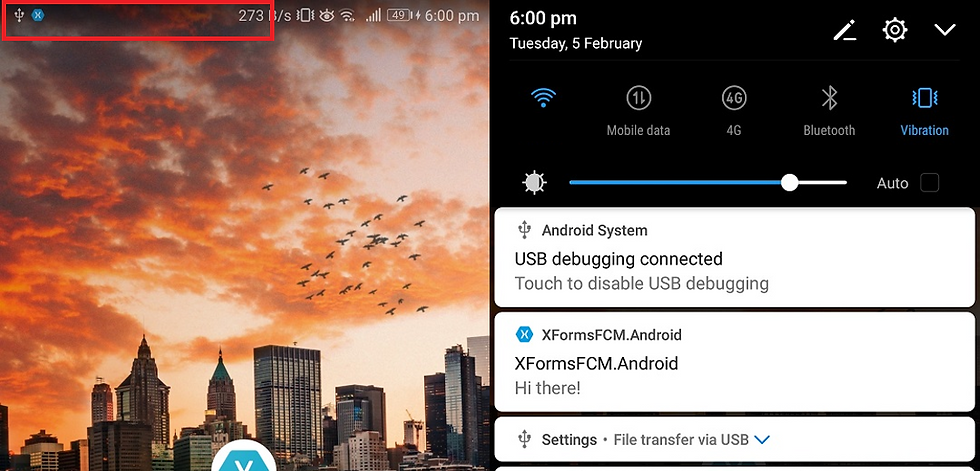
And here’s what the app looks like when is running.
Here’s the GitHub repo of this demo.
Try it yourself! Until next time. Happy coding!
Woah!! Such a piece of the nice information you have shared here, I have read the entire post and I must say that the information is very helpful for me.
http://www.hire-xamarin-developer.com/
http://hire-xamarin-developer.com/xamarin-development-company.html